useStyles()
useStyles()
is core hook which is used by the Stylo library to read styles from theme. It accepts StyleNames
& StyleNamespace
as its arguments. It then reads the styles defined for those StyleNames
under that StyleNamespace
in the theme, combines these styles into one & returns the final style, a standard React Native style object.
StyleNamespaces are used to organize the style definitions by logically grouping them together. This prevents the style name/style collisions, like Small
can have different style definitions for Text
& View
components. In the code snippet below TextStyles
, TextInputStyles
, ViewStyles
are the StyleNamespaces.
Stylo considers each React Native component as one StyleNamespace. To keep it simple & easily understandable, Stylo uses a pattern [ReactNativeComponentName]Styles
to name the StyleNamespace. The library uses following StyleNamespaces defined for each React Native component.
type TStyleNamespace =
| 'IconStyles'
| 'ImageBackgroundStyles'
| 'ImageStyles'
| 'SafeAreaViewStyles'
| 'ScrollViewStyles'
| 'ScrollViewContentContainerStyles'
| 'TextInputStyles'
| 'TextStyles'
| 'TouchableStyles'
| 'ViewStyles';
Note: New StyleNamespaces for remaining components will be added soon.
Stylo recommends to use the Stylish components & Styler hooks instead ofuseStyles()
hook. TheuseStyles()
hook is used inside all the Stylish components & Styler hooks. So technically, using only thisuseStyles()
hook any style definition defined in the theme can be accessed & applied to the components. In practice, one may not need to use theuseStyles()
hook directly.
Type definition
function useStyles<
TStyleProp,
TStyleName extends string,
TStyleNamespace extends string
>({ styleNamespace, styleNames }: {
styleNamespace: TStyleNamespace;
styleNames: TStyleName[];
}): StyleProp<TStyleProp>
TStyleProp
Standard React Native stye prop like TextStyle, ViewStyle etc.
TStyleName
StyleName type defined in the theme.
TStyleNamespace
StyleNamespace type defined in the theme.
Return type
Standard React Native style object. E.g. StyleProp<ViewStyle>
.
Argument
{
styleNamespace: TStyleNamespace;
styleNames: TStyleName[];
}
styleNamespace
(Required)
The StyleNamespace to be used which holds the style definitions for the StyleNames
supplied to the hook.
styleNames
(Required)
The StyleNames which define the styles.
Return value
Standard React Native style object. E.g. StyleProp<ViewStyle>
.
Usage
Below example shows the use of useStyles()
hook to create a styled card.
import React, { useRef } from 'react';
import { SafeAreaView, Text, TextStyle, TouchableOpacity, View, ViewStyle } from 'react-native';
import { useStyles } from 'react-native-stylo';
import { TTextStyle, TTouchableStyle, TViewStyle } from './stylo/themes/types';
const UseStylesShowCase: React.FC = () => {
const styles = useRef({
screen: useStyles<ViewStyle, TViewStyle>({
styleNamespace: 'ViewStyles',
styleNames: ['Screen'],
}),
screenHeader: useStyles<ViewStyle, TViewStyle>({
styleNamespace: 'ViewStyles',
styleNames: ['Screen.Header', 'Padding'],
}),
screenTitle: useStyles<TextStyle, TTextStyle>({
styleNamespace: 'TextStyles',
styleNames: ['H1', 'Margin.Top.Small', 'Margin.Bottom.Small'],
}),
screenBody: useStyles<ViewStyle, TViewStyle>({
styleNamespace: 'ViewStyles',
styleNames: ['Screen.Body', 'Padding'],
}),
card: useStyles<ViewStyle, TViewStyle>({
styleNamespace: 'ViewStyles',
styleNames: ['Border', 'Border.Radius', 'BackgroundColor.Alpha10', 'Margin.Bottom.Large'],
}),
cardHeader: useStyles<ViewStyle, TViewStyle>({
styleNamespace: 'ViewStyles',
styleNames: ['Padding.Top', 'Padding.Left', 'Padding.Right'],
}),
cardBody: useStyles<ViewStyle, TViewStyle>({
styleNamespace: 'ViewStyles',
styleNames: ['Padding'],
}),
cardFooter: useStyles<ViewStyle, TViewStyle>({
styleNamespace: 'ViewStyles',
styleNames: ['Padding', 'Border.Top'],
}),
cardTitle: useStyles<TextStyle, TTextStyle>({
styleNamespace: 'TextStyles',
styleNames: ['Large', 'Bold'],
}),
code: useStyles<TextStyle, TTextStyle>({
styleNamespace: 'TextStyles',
styleNames: ['Color.Grey8', 'Bold.Semi'],
}),
description: useStyles<TextStyle, TTextStyle>({
styleNamespace: 'TextStyles',
styleNames: ['Color.Grey9'],
}),
footerButton: useStyles<ViewStyle, TTouchableStyle>({
styleNamespace: 'TouchableStyles',
styleNames: ['Button', 'Border', 'Border.Color.Primary'],
}),
footerButtonText: useStyles<TextStyle, TTextStyle>({
styleNamespace: 'TextStyles',
styleNames: ['Color.Primary'],
}),
}).current;
return (
<View style={styles.screen}>
<SafeAreaView />
<View style={styles.screenHeader}>
<Text style={styles.screenTitle}>useStyles() hook</Text>
</View>
<View style={styles.screenBody}>
<View style={styles.card}>
<View style={styles.cardHeader}>
<Text style={styles.cardTitle}>Type definition</Text>
</View>
<View style={styles.cardBody}>
<Text style={styles.code}>
{
'function useStyles<TStyleProp, TStyleName extends string, TStyleNamespace extends string>({ styleNamespace, styleNames }: { styleNamespace: TStyleNamespace; styleNames: TStyleName[]; }): StyleProp<TStyleProp>'
}
</Text>
</View>
</View>
<View style={styles.card}>
<View style={styles.cardBody}>
<Text style={styles.description}>
useStyles() is main hook which is used by the Stylo library to
read styles from theme. It accepts StyleNames & optional
StyleNamespace as its arguments. It then reads the styles defined
for those StyleNames under that StyleNamespace in the theme,
combines these styles into one & returns the final style.
</Text>
</View>
<View style={styles.cardFooter}>
<TouchableOpacity style={styles.footerButton}>
<Text style={styles.footerButtonText}>Read more ...</Text>
</TouchableOpacity>
</View>
</View>
</View>
</View>
);
};
export default UseStylesShowCase;
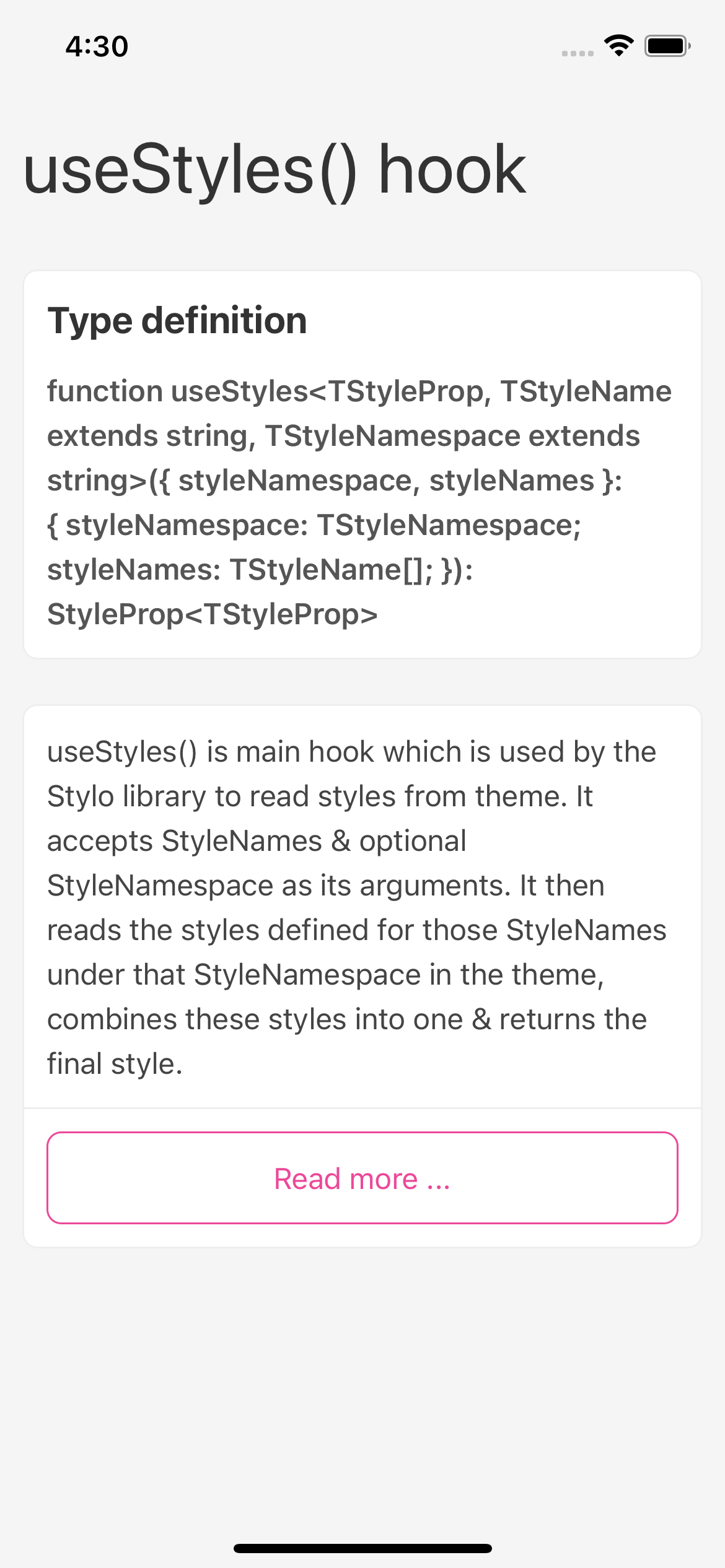